EXIF is ” a standard that specifies the formats for images, sound, and ancillary tags used by digital cameras (including smartphones), scanners and other systems handling image and sound files recorded by digital cameras.”(from Wikipedia)
When uploading or sharing the photos that you took, there might be a possibility that some personal information would expose to the public and thus cause some trouble. Therefore here is a way to delete the EXIF data with python.
First you need to install piexif (or readexif,pillow(PIL), etc., based on what you would like to use). Note that some tutorials use PIL, but apparently it only supports python 1.5.2–2.7 and was discontinued a decade ago, so please use Pillow instead.
$ pip install piexif
piexif.load:
“Returns exif data as a dictionary with the following keys: “0th”, “Exif”, “GPS”, “Interop”, “1st”, and “thumbnail”. “
import piexif
exif_dict = piexif.load('akb.jpg')
print(exif_dict.keys())
>>>dict_keys(['0th', 'Exif', 'GPS', 'Interop', '1st', 'thumbnail'])
Reference to piexif doc:https://piexif.readthedocs.io/en/latest/functions.html
exif_dict = piexif.load("foo.jpg")
thumbnail = exif_dict.pop("thumbnail")
if thumbnail is not None:
with open("thumbnail.jpg", "wb+") as f:
f.write(thumbnail)
for ifd_name in exif_dict:
print("\n{0} IFD:".format(ifd_name))
for key in exif_dict[ifd_name]:
try:
print(key, exif_dict[ifd_name][key][:10])
except:
print(key, exif_dict[ifd_name][key])
I used a photo that I took in Akihabara for example, using RICOH GRII.
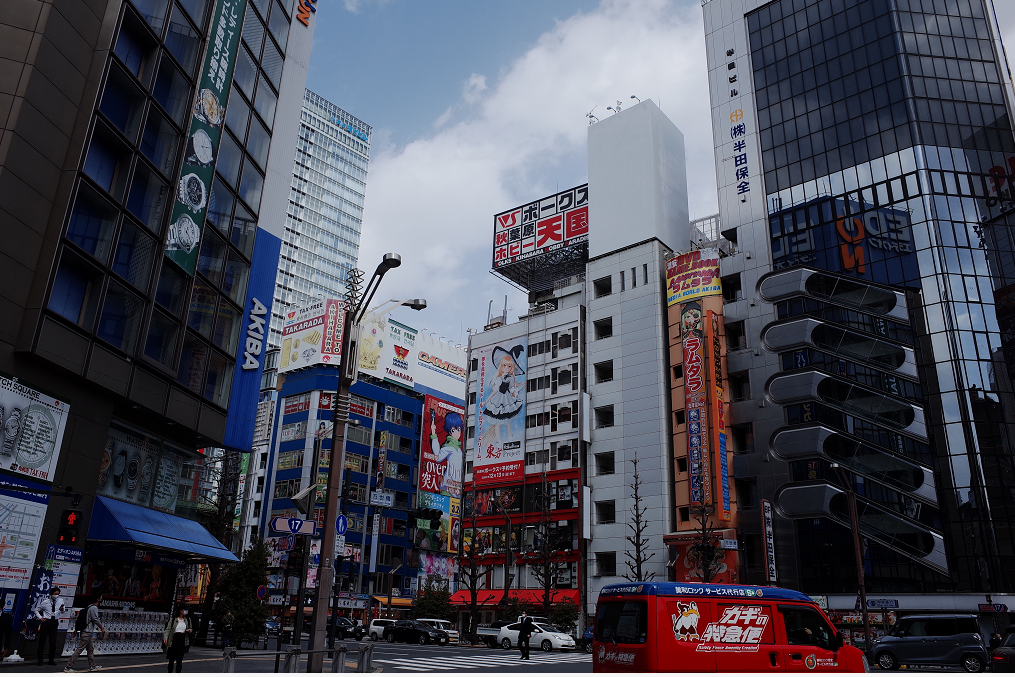
Here is the result that I got when running the sample code:
0th IFD:
270 b' '
271 b'RICOH IMAG'
272 b'GR II\x00\x00\x00\x00\x00'
274 1
282 (72, 1)
283 (72, 1)
296 2
305 b'GR Firmwar'
306 b'2021:03:30'
531 2
33432 b'*omitted*'
34665 454
34853 1114
50341 b'PrintIM\x0003'
Exif IFD:
33434 (10, 12500)
33437 (400, 100)
34850 2
34855 100
34864 1
36864 b'0230'
36867 b'2021:03:30'
36868 b'2021:03:30'
37121 b'\x01\x02\x03\x00'
37122 (320, 100)
37378 (40, 10)
37379 (86, 10)
37380 (-7, 10)
37381 (30, 10)
37383 5
37384 0
37385 16
37386 (183, 10)
37500 b'Ricoh\x00\x00\x00\x00A'
37510 b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'
40960 b'0100'
40961 1
40962 4928
40963 3264
40965 34048
41986 0
41987 0
41989 28
41990 0
41994 0
42032 b' '
42034 ((1830, 100), (1830, 100), (28, 10), (28, 10))
42035 b'RICOH IMAG'
42036 b'GR LENS '
GPS IFD:
0 (2, 3, 0, 0)
Interop IFD:
1 b'R98'
1st IFD:
259 6
282 (72, 1)
283 (72, 1)
296 2
513 34532
514 4498
To delete the exif data, simply use piexif.remove:
piexif.remove("akb.jpg")
for ifd_name in exif_dict:
for key in exif_dict[ifd_name]:
print(key,exif_dict[ifd_name][key][:10])
The result is now empty:
0th IFD:
Exif IFD:
GPS IFD:
Interop IFD:
1st IFD: